Introduction
JavaScript developer practices serve as a critical foundation in web development.
Its versatility powers both front-end and back-end applications.
Developers utilize JavaScript to create interactive websites that engage users.
Given the rise of web technologies, mastering JavaScript has become essential.
Adhering to best practices is key for developers.
It enhances performance, ensures maintainability, and improves collaboration among team members.
Well-written JavaScript code is easier to read and understand.
This efficiency fosters teamwork, especially in diverse environments like Nigeria.
This blog post aims to highlight essential JavaScript best practices for Nigerian developers.
By following these guidelines, developers can streamline their coding process.
This approach not only boosts productivity but also enhances the quality of their work.
Commitment to best practices leads to a better understanding of JavaScript fundamentals.
Moreover, developers can share their knowledge with peers, creating a more robust programming community in Nigeria.
Engaging with best practices connects local developers with global trends.
This connection can spark innovation and growth within the industry.
As we dive deeper into this topic, let’s explore the most effective practices to implement.
Emphasizing clean code principles, regular testing, and effective version control will position developers for success.
Staying updated with JavaScript frameworks and libraries is equally crucial.
Understanding new tools can enhance your development process significantly.
Basically, embracing these best practices will elevate the coding skills of Nigerian developers.
As the tech landscape evolves, adapting to industry standards will be paramount.
This commitment will ensure that developers contribute meaningfully to the global tech community.
Understanding JavaScript Syntax and Semantics
JavaScript is a powerful language with specific syntax rules and semantics.
To write effective code, developers must grasp these principles.
Here, we will discuss the critical aspects of JavaScript syntax, the importance of variable naming, and the role of semicolons.
Overview of Basic JavaScript Syntax Rules
JavaScript syntax involves the set of rules that defines a correctly structured program.
Understanding these rules helps you write code that is easy to read and maintain.
Here are essential elements of JavaScript syntax:
- Comments: Use single-line (//) or multi-line (/* … */) comments to document your code.
- Variables: Declare variables using let, const, or var.
Using let and const is recommended for modern development. - Data Types: JavaScript supports several data types, including strings, numbers, booleans, null, undefined, and objects.
- Operators: Use arithmetic, comparison, logical, and assignment operators according to your program’s needs.
- Control Structures: Implement conditional statements (if, else, switch) and loops (for, while, do-while) to control the flow of your code.
- Functions: Define functions using function declarations, function expressions, or arrow functions.
Functions allow you to encapsulate reusable logic.
By mastering these syntax rules, you enhance your ability to write clean, effective JavaScript code, vital for any developer.
Importance of Using Descriptive Variable Names and Consistent Naming Conventions
The choice of variable names significantly impacts the readability and maintainability of your code.
Descriptive names convey meaning, making your code easier to understand.
Here are some best practices for naming variables:
- Be Descriptive: Choose names that describe the purpose of the variable.
For instance, useemployeeCount
instead ofec
. - Use Camel Case: For multi-word variable names, use camel case, such as
customerOrder
.
This convention enhances clarity. - Avoid Reserved Keywords: Do not use JavaScript reserved words (like
class
,function
, orvar
) as variable names. - Be Consistent: Maintain a consistent naming convention throughout your code. Consistency improves readability.
- Indicate Constants: When using constants, consider using uppercase letters with underscores. For example,
MAX_USERS
.
Using descriptive variable names fosters a clearer understanding of code, which is crucial for collaboration and future maintenance.
Implications of Semicolon Usage
In JavaScript, semicolons serve as statement terminators.
While many developers often ignore them, understanding their importance is crucial.
Here are notable points about semicolon usage:
- Preventing Errors: Omitting semicolons can lead to unexpected errors.
JavaScript performs automatic semicolon insertion (ASI), which may not always behave as intended. - Code Clarity: Using semicolons at the end of statements improves clarity.
It makes it easier to differentiate between separate statements. - Consistency: By consistently using semicolons, you develop a habit that promotes best coding practices.
This consistency helps avoid confusion. - Minification and Optimization: When minifying JavaScript for production, semicolons prevent errors.
They ensure that the code remains functional after compression. - When to Omit: Sometimes omitting semicolons is acceptable, especially with single-line statements.
However, remain cautious in complex scenarios.
By paying attention to semicolon usage, you mitigate potential errors and enhance your code’s overall quality.
Understanding JavaScript syntax and semantics is the foundation for any developer.
By adhering to best practices, you improve code readability and maintainability.
Descriptive variable names and proper semicolon usage are critical aspects of this journey.
Embrace these principles for effective JavaScript programming in your projects.
Using Strict Mode
JavaScript provides a feature known as strict mode that helps developers write cleaner, more secure code.
Strict mode enforces stricter parsing and error handling on your JavaScript code.
By using strict mode, developers can avoid certain common pitfalls and write more reliable code.
Unlock Your Unique Tech Path
Get expert tech consulting tailored just for you. Receive personalized advice and solutions within 1-3 business days.
Get StartedWhat is Strict Mode?
Strict mode is a way to opt in to a restricted variant of JavaScript.
It activates a different set of rules that help ensure better programming practices.
This mode helps identify potential bugs early in the development process.
When you apply strict mode, JavaScript performs additional checks and throws errors for unsafe actions.
It can prevent you from using certain language features that might lead to errors.
With strict mode, you develop more maintainable and predictable code.
Benefits of Using Strict Mode
- Prevents accidental global variables: Without strict mode, assigning a value to an undeclared variable creates a global variable.
Strict mode throws an error if you attempt this, preventing unexpected behavior. - Disallows duplicate parameter names: In strict mode, defining functions with duplicate parameter names results in an error.
This eliminates confusion and ensures code clarity. - Secures this context: In strict mode, the value of “this” inside a function that is not called on an object is undefined.
This prevents common pitfalls linked to the “this” keyword. - Enforces variable declaration: Strict mode requires explicit declaration of all variables using “let”, “const”, or “var”.
This reduces the likelihood of creating unintentional global variables. - Enhances security: Strict mode restricts the use of features that may lead to security vulnerabilities, making your applications more robust.
How to Enable Strict Mode
Enabling strict mode is straightforward.
You can apply it either globally or locally in your code.
To enable strict mode globally, place the following statement at the beginning of your JavaScript file:
"use strict";
Alternatively, you can apply strict mode to individual functions.
Just include the same statement at the top of the function:
function myFunction() {
"use strict";
// Your code here
}
Common Pitfalls Avoided by Using Strict Mode
Strict mode helps developers avoid various common mistakes in JavaScript.
Here are some pitfalls that strict mode mitigates:
- Using undeclared variables: When you do not declare a variable properly, it can leak into the global scope.
Strict mode throws an error in such cases. - Accidental creation of global variables: Assigning a value to a variable without declaring it leads to the creation of a global variable.
Strict mode prevents this situation by throwing an error. - Misuse of the “this” keyword: In non-strict mode, calling a function without an object context sets “this” to the global object.
Strict mode prevents misunderstandings by making “this” undefined in such cases. - Duplicate parameter names: Defining a function with duplicate parameter names can lead to unexpected behavior.
Strict mode eliminates this possibility by throwing an error. - Octal literals: JavaScript allows octal literals, which can be confusing.
Strict mode disallows them, promoting clarity in numeric representations.
Incorporating strict mode into your JavaScript code can significantly improve your programming practices.
By enforcing stricter rules, strict mode helps identify errors that could lead to bugs or security issues.
Moreover, strict mode encourages better coding standards.
It promotes clarity and maintainability by making developers conscious of their coding habits.
By avoiding common pitfalls, developers can minimize unintended consequences.
As a Nigerian developer, embracing strict mode is a step towards writing high-quality, reliable JavaScript code.
By understanding the benefits and implementation of strict mode, you contribute to the development of robust web applications.
In fact, using strict mode simplifies debugging while enhancing code reliability.
It also fosters a better understanding of JavaScript rules and behavior.
Therefore, always consider enabling strict mode in your JavaScript projects.
Read: Best Coding Practices Every Nigerian Developer Should Follow
Writing Modular Code
Writing modular code transforms your development experience.
It boosts both scalability and readability in JavaScript projects.
Developers, especially in Nigeria, can take significant advantage of this approach.
Modular code allows teams to work simultaneously on different parts of applications.
The Importance of Modular Code
- Enhanced Readability: Modular code breaks down complex systems into manageable blocks.
Each module performs a specific function, making it easier to understand. - Improved Maintainability: When projects grow, maintaining a single large file becomes challenging.
Modular design allows developers to update individual components without affecting the whole system. - Reusability: Developers can reuse modules across multiple projects.
This practice saves time and effort, allowing for faster development cycles. - Team Collaboration: Different teams can work on separate modules.
Parallel development increases productivity and enhances project delivery timelines. - Scalability: Modular code simplifies adding new features.
New modules can integrate seamlessly without overhauling existing structures.
Introduction to ES6 Modules
ES6 introduced a formal module system into JavaScript.
This system provides a clear way to structure your JavaScript applications.
You can create modules to handle various functionalities, which simplifies your project architecture.
Modules in ES6 use the export
and import
statements.
These keywords allow you to share and load code between different files.
Here’s a fundamental example:
Unlock Premium Source Code for Your Projects!
Accelerate your development with our expert-crafted, reusable source code. Perfect for e-commerce, blogs, and portfolios. Study, modify, and build like a pro. Exclusive to Nigeria Coding Academy!
Get Code// math.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
// main.js
import { add, subtract } from './math.js';
console.log(add(5, 3)); // 8
console.log(subtract(5, 3)); // 2
In this example, mathematical functions are organized into the math.js
file.
The main.js
file imports these functions, promoting modularity.
Implementing ES6 Modules
- Creating Modules: Begin by creating JavaScript files that contain your functions or classes.
Each file acts as a module. - Using
export
: Decide what functionality to expose from your module.
Use theexport
keyword for those elements. - Importing Modules: Use the
import
keyword to bring in functionality from other modules.
Specify the module path accurately to avoid errors. - Default Exports: You can also export a single value as the default.
Useexport default
to allow simple imports.
Best Practices for Organizing Files and Modules
Organizing your modules and files is crucial for maintaining a clean codebase.
Here are some best practices to follow:
- Use a Consistent Naming Convention: Adopt a naming convention for files and folders.
Consistency facilitates easier navigation. - Group Related Modules: Create directories to group related modules together.
For instance, store all utility functions in autils
folder. - Create an Index File: Use an
index.js
file in a directory to re-export modules.
This practice simplifies your import statements. - Keep Modules Focused: Each module should focus on a single responsibility.
This clarity enhances code maintainability. - Limit Module Size: Try to keep modules small and concise.
Large modules can become hard to maintain and understand. - Document Your Code: Provide comments and documentation for your modules.
Clear documentation helps other developers comprehend your code easily. - Implement Automated Testing: Ensure all modules are testable.
Write unit tests for each module to improve reliability.
Incorporating these practices enhances your code quality significantly.
Doing so can lead to a smoother development process, especially as your projects grow in complexity.
Practical Example of Modular Code Structure
Consider a simple web application.
You might structure your files as follows:
src/
├── index.html
├── css/
│ └── styles.css
├── js/
│ ├── app.js
│ ├── modules/
│ │ ├── math.js
│ │ ├── user.js
│ │ ├── api.js
│ └── utils/
│ └── helpers.js
In this structure, the js
folder contains all JavaScript files.
Under modules
, you can find files that manage different functionalities.
The utils
folder contains helper functions to keep things organized.
Writing modular code is essential in modern JavaScript development.
By embracing ES6 modules and adhering to best practices, developers can create scalable and maintainable applications.
Doing so fosters collaboration, simplifies debugging, and enhances code readability.
For Nigerian developers, mastering modular code represents a vital step towards professional growth and project success.
As you continue your journey in JavaScript development, remember the importance of structure.
Invest time in organizing your code, and you will feel the positive impacts on your workflow.
Read: 5 Creative Python Projects Nigerians Can Build to Get Hired
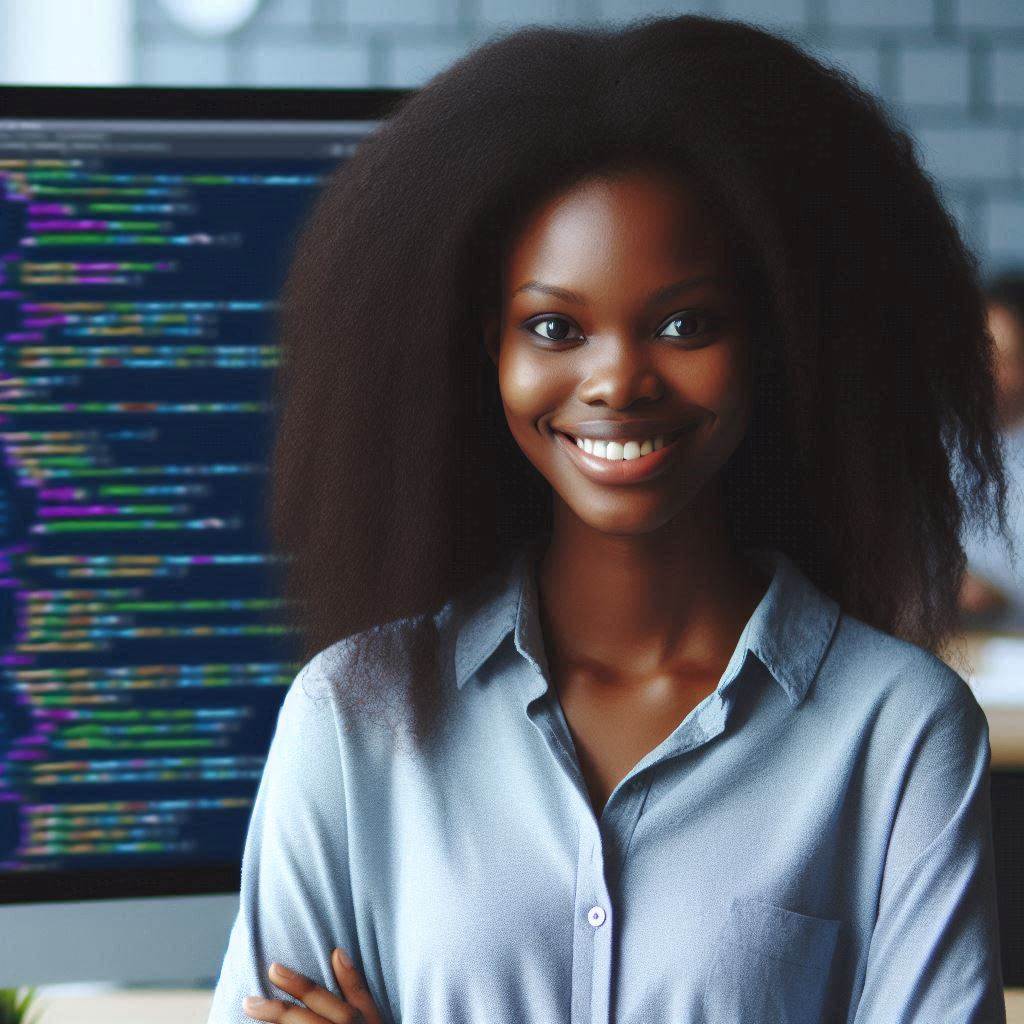
Leveraging Asynchronous Programming
Understanding Asynchronous Programming
Asynchronous programming enables JavaScript to process tasks without blocking the main thread.
This approach enhances performance and user experience.
In a traditional synchronous model, code execution occurs linearly.
This means that one line must fully execute before the next begins.
If a task takes too long, the user interface freezes.
Asynchronous programming resolves these issues by allowing the browser to manage tasks concurrently.
To grasp asynchronous programming, it is essential to understand three core concepts: callbacks, promises, and async/await.
Callbacks
A callback is a function that a program passes as an argument to another function.
This allows code to run after another task completes.
While callbacks are simple, they can lead to complex structures, often referred to as “callback hell.”
Here’s a simple example of a callback:
function fetchData(callback) {
setTimeout(() => {
let data = 'Data fetched';
callback(data);
}, 1000);
}
fetchData((data) => {
console.log(data);
});
Though this approach is straightforward, nesting callbacks can create unreadable code.
Promises
Promises improve upon callbacks by offering better readability and error handling.
A promise can be in one of three states: pending, fulfilled, or rejected.
You initiate a promise by calling its constructor:
let fetchData = new Promise((resolve, reject) => {
setTimeout(() => {
let success = true;
if (success) {
resolve('Data fetched successfully');
} else {
reject('Error fetching data');
}
}, 1000);
});
fetchData
.then((result) => console.log(result))
.catch((error) => console.error(error));
Promises promote cleaner code layouts and separate asynchronous operations from their handling.
This separation enhances maintenance and debugging.
Async/Await
The async/await syntax further simplifies asynchronous programming.
By using the async
keyword before a function, you enable the use of await
within that function.
The await
keyword pauses the execution of the async function until a promise resolves:
async function fetchData() {
try {
let data = await new Promise((resolve) => {
setTimeout(() => resolve('Data fetched with async/await'), 1000);
});
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
The async/await syntax leads to clearer and more concise code.
It makes error handling easier using try/catch blocks.
Best Practices for Handling Asynchronous Code
Implementing asynchronous programming effectively enhances performance and user experience.
Here’s a list of best practices:
- Use Promises and Async/Await: Favor these patterns over callbacks.
They improve readability and error handling. - Handle Errors: Always use
catch
with promises ortry/catch
with async/await to manage exceptions. - Limit Concurrent Requests: Avoid overwhelming servers or browsers by controlling how many asynchronous processes run simultaneously.
- Use Throttling and Debouncing: These techniques help manage events like scrolling or resizing without excessive function calls.
- Utilize Loading Indicators: Provide visual feedback to users when data is being fetched.
This reduces frustration. - Cache Responses: Caching frequently accessed data minimizes redundant network requests, enhancing performance.
- Optimize Network Requests: Compress data and limit payload sizes to improve load times.
- Test Asynchronous Code: Employ libraries like Jest or Mocha to ensure your asynchronous functions behave as expected.
Common Libraries and Tools
Several libraries and tools can simplify asynchronous programming in JavaScript.
Below is a list of popular options:
- Axios: A promise-based HTTP client for making requests. It simplifies data fetching.
- Fetch API: A built-in web API that allows easy and flexible AJAX requests.
It utilizes promises. - RxJS: A library for reactive programming using observables.
It offers powerful tools for handling asynchronous data streams. - async.js: A utility module that provides straightforward methods for working with asynchronous JavaScript.
- Bluebird: A fully-featured promise library that offers advanced features like cancellation and timing.
Mastering asynchronous programming is vital for every Nigerian developer.
Understanding callbacks, promises, and async/await equips developers with essential skills.
Implementing best practices enhances performance and boosts user experience.
Leveraging common libraries streamlines development.
By adopting these principles, developers can create faster, more responsive web applications.
Read: Building FinTech Apps for Nigerians: Flutter Made Simple
Effective Error Handling
Importance of Error Handling in Building Robust Applications
Error handling plays a crucial role in software development.
Developers must write code that not only functions properly but also gracefully handles unexpected situations.
When errors occur, they can significantly impact user experience.
Users encounter frustrating situations when applications crash.
Proper error management minimizes these scenarios, contributing to a smoother experience.
Moreover, error handling helps in identifying and diagnosing issues promptly.
Mistakes or bugs in code are inevitable.
When developers anticipate possible errors, they can address them before they cause major problems.
Well-managed error handling allows for seamless debugging, making it easier to maintain and update code.
Techniques for Error Handling
JavaScript provides several techniques for effective error handling.
Here are three fundamental methods:
- Try/Catch Blocks: The try block contains code that could throw an error.
If an error occurs, the catch block executes.
This method allows developers to manage exceptions without disrupting the application’s flow. - Custom Error Types: Creating custom error types helps differentiate between various errors.
For example, you can create specific error classes for user input, network issues, or data processing.
This distinction allows for more precise error handling. - Logging: Keeping a log of errors is vital for understanding application health.
Logging aids in tracking issues that require immediate attention.
Developers can use a combination of console logging and external monitoring tools for thorough oversight.
Using these techniques, developers can improve the overall stability and reliability of their applications.
Creating User-Friendly Error Messages
User-friendly error messages enhance the user experience significantly.
Here are some strategies for crafting effective messages:
- Clarity: Ensure that the error message is straightforward.
Avoid technical jargon that might confuse users.
Simple language helps users understand what went wrong. - Actionable Guidance: Offer suggestions on how to resolve the issue.
For example, tell users if they need to retry a task or check their internet connection.
This step helps users take corrective actions quickly. - Politeness: Maintain a courteous tone in messages.
Apologize for the inconvenience and express a willingness to help.
A polite message can mitigate frustration. - Consistency: Keep error messages consistent across your application.
Users prefer familiarity.
Consistency creates a smoother experience while interacting with your application. - Support Information: Provide users with resources for further assistance.
This could be links to FAQs, contact details, or a support chat option.
Giving users more options reduces frustration.
By focusing on these aspects, developers can create a more pleasant and supportive user experience.
Implementing Error Handling Best Practices
To further enhance error handling in applications, developers should follow these best practices:
- Anticipate Errors: Always expect potential errors at various points in code execution.
Proper anticipation leads to proactive solutions - Centralized Error Handling: Use centralized error management to streamline responses.
This practice fosters consistency in how errors are processed. - Analyze Error Data: Regularly analyze logged error data.
Understanding common issues can highlight areas for improvement and future prevention. - Testing Error Handling: Include error scenarios in your testing strategy.
Using unit tests and integration tests ensures that error handling mechanisms work as intended. - Use Promises or Async/Await: When dealing with asynchronous code, utilize promises or the async/await syntax for error handling.
This approach avoids callback hell and improves readability.
Implementing these best practices strengthens the resilience and maintainability of your applications.
Effective error handling is essential for creating robust JavaScript applications.
By understanding the importance, techniques, and best practices of error handling, Nigerian developers can build improved user experiences.
Developers need to leverage tools and methods that empower their applications to handle errors gracefully.
Ultimately, meticulous error handling fosters trust and satisfaction among users, leading to better software solutions.
Read: 10 Exciting Programming Projects to Level Up in Nigeria
Optimizing Performance
Optimizing JavaScript performance is essential for creating a fluid user experience.
Poor performance can frustrate users and drive them away.
As Nigerian developers, we must implement strategies to enhance load times and responsiveness.
Below, we explore key optimization strategies, tools for profiling performance, and the differences between synchronous and asynchronous loading techniques.
Key Strategies for Optimizing JavaScript Performance
Here are some effective strategies developers can use to optimize JavaScript performance:
- Lazy Loading: Load content only when necessary.
This technique improves initial load times. - Code Minification: Reduce the size of your JavaScript files by removing unnecessary spaces and comments.
Minified files load faster. - Debouncing and Throttling: Use these techniques to limit the rate of function execution.
This is particularly useful for events like scrolling and resizing. - Tree Shaking: Eliminate unused code from your final bundle.
This leads to smaller file sizes. - Using Web Workers: Offload heavy computations to background threads to keep your main thread responsive.
- Caching: Cache frequently accessed data to minimize network requests and enhance loading speeds.
Tools and Techniques for Profiling JavaScript Performance
Profiling is critical to identifying performance bottlenecks.
Here are relevant tools and techniques:
- Chrome DevTools: Inspect performance with the Performance tab.
Use it to analyze loading times and rendering. - Lighthouse: This tool audits web apps and provides insights on performance metrics and improvements.
- PageSpeed Insights: Google’s tool provides analysis and actionable recommendations to boost performance.
- WebPageTest: Use this tool to conduct detailed tests for loading times and view the waterfall charts.
- JSPerf: Create benchmark tests for different code snippets to compare their performance directly.
Comparison of Synchronous vs Asynchronous Loading Techniques
Understanding loading techniques can drastically affect your app’s performance.
Here are the key differences:
Synchronous Loading
Synchronous loading happens in sequence.
This means:
- The browser must fully load one resource before loading the next.
- It can significantly delay page rendering and block user interaction.
- Synchronous scripts can lead to a slower overall user experience.
- Developers often add synchronous JavaScript in the
<head>
section, which leads to render blocking.
Asynchronous Loading
Asynchronous loading allows other resources to load simultaneously.
Key points include:
- The browser fetches the script in parallel with other resources without freezing the UI.
- This method enhances user experience by allowing immediate rendering of content.
- Asynchronous scripts can be added at the bottom of the
<body>
or with theasync
attribute. - Using the
defer
attribute ensures scripts execute in order without blocking rendering.
Performance optimization is vital for JavaScript applications’ success.
By employing techniques such as lazy loading and code minification, developers can enhance user experience.
Tools like Chrome DevTools and Lighthouse offer insights for profiling performance effectively.
Furthermore, understanding the differences between synchronous and asynchronous loading can lead to significant improvements.
Nigerian developers must adopt these practices to build fast, efficient, and responsive applications.
Security Best Practices
Common Security Vulnerabilities in JavaScript Applications
JavaScript applications face numerous security challenges.
Understanding these vulnerabilities is crucial for developers.
Let’s examine some common security vulnerabilities below:
- Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages.
This can lead to data theft and account hijacking. - Cross-Site Request Forgery (CSRF): CSRF exploits user sessions.
It tricks users into executing unwanted actions on web applications. - SQL Injection: Attackers insert malicious SQL queries.
This can lead to unauthorized data access and manipulation. - Insecure Direct Object References (IDOR): Attackers gain access to unauthorized data.
They do this by manipulating input parameters. - Server-Side Request Forgery (SSRF): Attackers make unauthorized requests to internal services.
This can expose sensitive data.
Best Practices to Mitigate Security Risks
Developing secure JavaScript applications requires vigilance and proactive measures.
By implementing best practices, developers can significantly reduce security risks.
Below are essential practices to follow:
- Input Validation: Always validate and sanitize user input.
Never trust data from untrusted sources. - Output Encoding: Encode data before displaying it.
This prevents XSS attacks by ensuring that user input does not execute as code. - Use Secure API Calls: Employ HTTPS for all API communications.
This protects data during transmission and prevents interception. - Implement Content Security Policy (CSP): Use CSP to control which resources can be loaded.
This helps mitigate XSS and data injection attacks. - Utilize Security Libraries: Incorporate libraries designed for security.
Tools like DOMPurify can help sanitize HTML input effectively. - Regular Security Reviews: Conduct periodic security audits of your code.
Identify potential vulnerabilities and address them promptly.
Importance of Keeping Libraries and Frameworks Up to Date
Maintaining up-to-date libraries and frameworks is essential for security.
Older versions often have known vulnerabilities.
Here’s why regular updates matter:
- Patch Vulnerabilities: Developers frequently release updates to fix security flaws.
Regularly updating ensures you benefit from these patches. - Benefit from New Features: Updated libraries often include new features.
These features can enhance security and improve performance. - Community Support: Using the latest versions allows you to access community support easily.
Developers often discuss bugs and exploits in current versions. - Compliance: Keeping libraries updated ensures compliance with security standards.
Many regulations require businesses to maintain security best practices.
In essence, developers must prioritize security in JavaScript applications.
By understanding common vulnerabilities and following best practices, you can build safer applications.
Keeping libraries up to date is equally vital in safeguarding against threats.
With diligence and a proactive approach, Nigerian developers can significantly enhance application security.
Conclusion
Understanding essential JavaScript best practices is vital for every Nigerian developer.
First, use meaningful variable names that enhance code readability.
Avoid vague abbreviations and instead choose names that describe the purpose.
This practice greatly aids collaboration and future project maintenance.
Second, prioritize modular code structure.
Break your code into functions and modules, promoting reusability and easier debugging.
Clean, modular code also allows others to understand your work faster.
Third, embrace modern JavaScript features.
Utilize ES6 syntax like arrow functions and destructuring to write concise and effective code.
Familiarity with the latest updates keeps your skills relevant in a rapidly changing landscape.
Next, manage errors gracefully. Implement try-catch blocks to handle exceptions seamlessly.
Error handling ensures a better user experience and maintains application stability.
Additionally, always keep performance in mind.
Avoid unnecessary loops and heavy computations, especially for large data sets.
Instead, leverage built-in JavaScript methods that enhance efficiency.
Furthermore, invest time in testing your code.
Use frameworks like Jasmine or Mocha to automate testing.
This investment can significantly reduce bugs and improve code reliability.
Adopting these practices not only improves your coding skills but also enhances your employability.
As you implement these best practices, you foster better coding habits and an environment conducive to professional growth.
Finally, engage with your community.
Join local development groups or online forums to share knowledge and experiences with fellow developers.
Continuous learning and sharing resources will enhance your skills and contribute to the broader tech ecosystem in Nigeria.
In short, commit to embracing these JavaScript best practices today.
Your growth as a developer hinges on your commitment to learning and applying these principles.
Keep pushing the boundaries of your knowledge and skills in JavaScript.